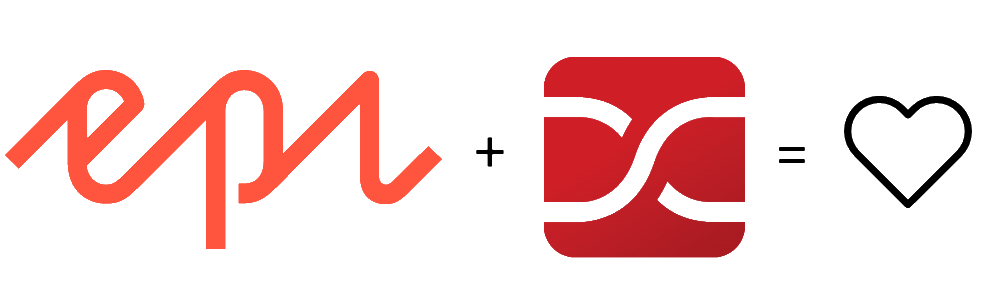
Episerver CMS + AutoMapper
Published:
Here is a short example of how to properly setup AutoMapper (opens new window) 5.1 in Episerver CMS (opens new window) 9 with dependency injection.
# Configuration
Start by installing nuget package AutoMapper.
Create a class “AutoMapperConfig” in the “Infrastructure”-folder. (Where Config files are located.)
public static class AutoMapperConfig
{
public static IMapper CreateIMapper()
{
var mapperConfiguration = new MapperConfiguration(cfg => cfg.AddProfiles(System.Reflection.Assembly.GetExecutingAssembly()));
return mapperConfiguration.CreateMapper();
}
}
This will “automagicaly” load all classes in project with base class “AutoMapper.Profiles”
Add this line to the ConfigureContainer Method in DependencyResolverInitialization class.
private static void ConfigureContainer(ConfigurationExpression container)
{
...
container.For<AutoMapper.IMapper>().Use(AutoMapperConfig.CreateIMapper());
...
}
Simple AutoMapper profile …that will be loaded automagicaly.
public class ModelMapping : Profile
{
public ModelMapping()
{
CreateMap<ModelDto, SiteModel>()
.ForMember(dest => dest.Text, opt => opt.MapFrom(src => src.Value));
}
}
Simple PageController using IMapper
public class SimplePageController : PageController<SimplePage>
{
private readonly AutoMapper.IMapper _mapper;
public SearchController(AutoMapper.IMapper mapper)
{
_mapper = mapper;
}
public ActionResult Index(SimplePage currentPage)
{
var viewModel = new SimplePageViewModel(currentPage);
viewModel.SiteModel = _mapper.Map<SiteModel>( /* insert modelDto */ )
return View(viewModel)
}
}
Finish! I’ve been using AutoMapper for a few weeks now and it has shown it self to be really useful. Definitely a tool worth some time to get to know!